In this blog we will learn Salesforce flow and how it is similar to apex coding.
Salesforce flow is a declarative way (Drag & Drop) of implementing an automation that is required for a business process alternative to code.
Blog covers types of flows, elements, resources and good comparison with a sample apex code.
Salesforce flow is a very powerful tool for admins/developers along with process builders and workflows. New admins/developers who are learning the Salesforce platform should put Salesforce flow as priority in their learning list.
Where do you find Salesforce Flow to start building one?
You should be Admin for an org to build salesforce flow, If you are admin please follow this path.
Go to “Setup” —-> Be on the “Home” tab —> Place the cursor on “Quick Find” —> Type “Flow”
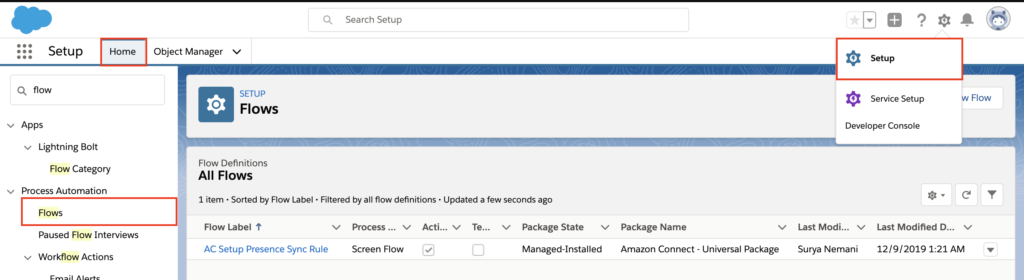
Types of Flows?
We have different types in flow for different automation use cases. Salesforce is also improving their flows greatly and providing more options in future.

Here are the types: Click on “New Flow” you will find above screen.
- Screen Flow: In this flow you provide screens to your users to guide them to get information which is required to launch operations based on their input.
- Record – After Save Updates: Very similar to auto launch flow but gives extra options, if you want to update/create the records before it has been saved to database or after it has been saved to database. Most likely you will find this to be very similar to Apex Trigger events before insert/update/delete and after insert/update/delete.

- Scheduled Flow: Schedule Jobs are only confined to developers until now. Scheduled flow will help you in achieving this by declarative development. Very similar to apex scheduled jobs
- Platform Event Flow: Listening to platform events and doing certain CRED operations is what this flow will help you achieve. Let’s say you have CTI configured your salesforce and now you would like to listen to a call platform event and invoke another apex action.
- Auto Launch Flow: Flow to perform CRED operations similar to Record after save flow without extra options.
Flows can be called via process builder, Auto launch flow will be called via process builder when where you define the criteria to launch the flow.
Deep dive in to Elements & Resources of flow:
When you select a type of flow and click next you will enter into a screen like below. This is where you will find tools and a canvas to build your logic.
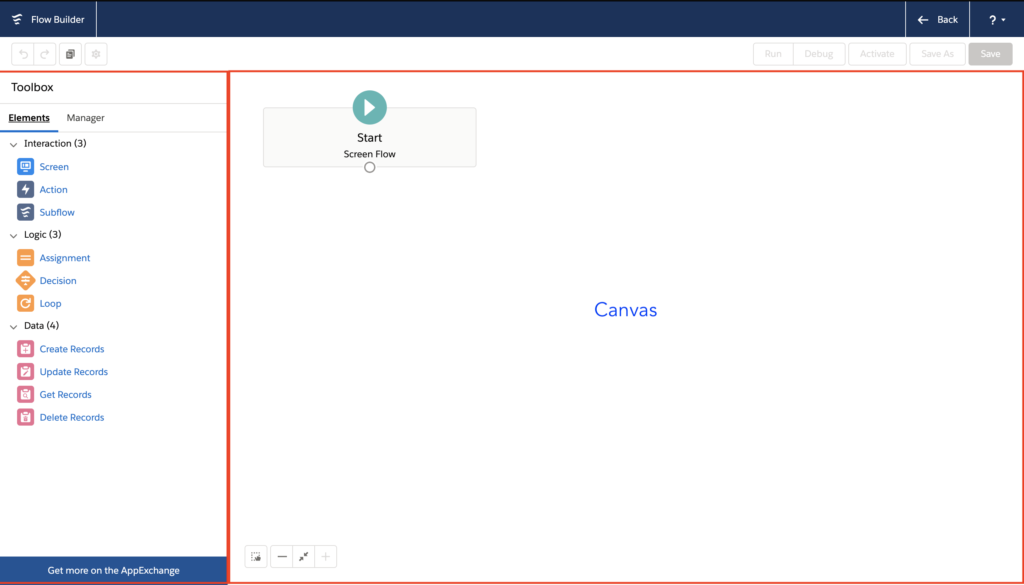
As you can see in the above picture, canvas is where you build step by step process. Drag and drop these elements into the canvas to build.
When your building flows by drag and dropping elements, you will be automatically creating resources which are organized in the “Manager” section.
Resources are your variables, data and other materials which will help in achieving your automation process.
Understanding layers of elements & how can I relate them to apex code:
Interactions:
- Screen: This will be only available when you select screen flow. You can build a screen by drag & drop in to the canvas and you will find options to place some data types.

- Actions: In this interaction element you can call Apex methods by making them invokable and set the variable and execute them. You can also trigger email alerts.

- Sub-flow: You can call other flows by using sub-flow elements.
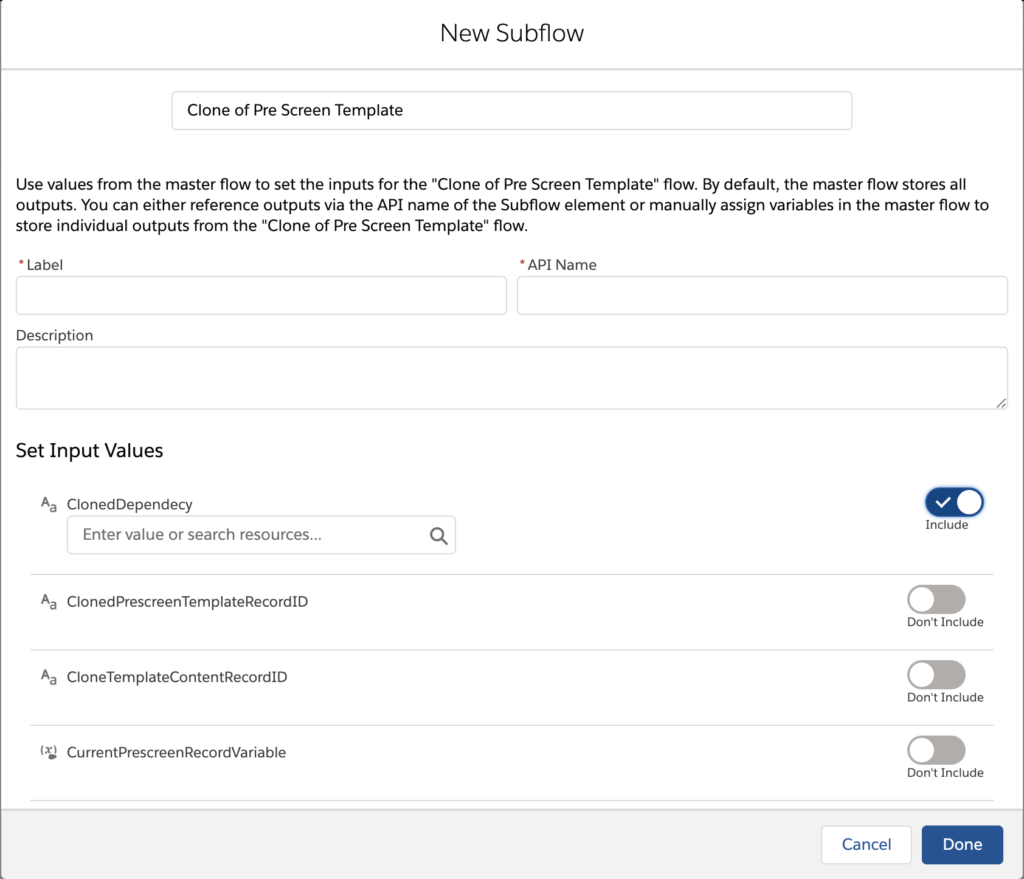
Logic:

- Assignment: Remember when you assign variables with a value within your Apex code. It has a similar function here.
- You can assign values to a variable
- You can assign values to record fields (Single Record Collection)
- You can assign add/remove records in record collection variable (Record Collection)
In the below code snippet, you can observe that a variable has been declared and assigned with a value. Now, let’s see how it is similar in Assignments of flow.
String strName = 'My String'; //String variable declaration Integer myInteger = 1; //Integer variable declaration Boolean mtBoolean = true; //Boolean variable declaration

Operator value has more options based on the variable type.
{!myInteger} – this is variable which is a resource that has to be created like a record and the screen looks something like this:

While you are creating resources you can select resource type, there are few options in this and will be explained in my next blog or can get materials from salesforce.
- Data Type: Variable data type is defined, we also have record as data type to store data of specific objects.
- API Name: Name what you give to your variable.
- Allow Multiple Values: Converts a variable into a collection variable where multiple values can be held, this can be used later in a Loop to iterate over the collections.
- Availability Outside the flow: Here you define if a variable will be available outside the flow that your building and how ?
- If it is available for input, it will be used as an input variable outside the flow that you are building.
- If it is available for output which means final results which are stored in variables will be available as output to trigger another flow.

- Loop: Remember the below code snippet where you use the “for” loop to iterate over collection of items and for each collection you perform an operation.
for (APEX_Invoice__c objInvoice: PaidInvoiceNumberList) { // Condition to check the current record in context values if (objInvoice.APEX_Status__c == 'Paid') { // current record on which loop is iterating System.debug('Value of Current Record on which Loop is iterating is'+objInvoice); // if Status value is paid then it will the invoice number into List of String InvoiceNumberList.add(objInvoice.Name); } }

Loop will have a collection and then a variable where each record from the collection is taken. Now this single record variable will hold one record at a time.
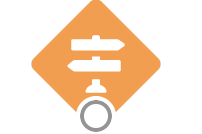
- Decisions: Classic if/else statements that can direct the operations that has to be executed based on certain condition
if boolean_expression { /* statement(s) will execute if the boolean expression is true */ } else { /* statement(s) will execute if the boolean expression is false */ }
But in flow’s decision element along with your if else statements, there is a default outcome statement, where when none of the criteria is met it will be executed. This is how it might look in full conditions.


Data:
Data in flows are very similar to your Apex SOQL operations like getting data and later you have your DML operations like Update, Delete, Create.
Data can be collected in with specific conditions or just get all the data and the same applies to your DML operations like Update, Delete, Create.

Re-Imagining Apex code in Salesforce flow:
We will take a piece of code and re-imagine that same code in flow. I will be using “Auto launch flow”.
// Initializing the custom object records list to store the Invoice Records created today
List PaidInvoiceNumberList = new List ();
// SOQL query which will fetch the invoice records which has been created today
PaidInvoiceNumberList = [SELECT Id,Name, APEX_Status__c,Paid_Date__c FROM APEX_Invoice__c WHERE
CreatedDate = System.today()];
// List to store the Invoice Number of Paid invoices
List InvoiceNumberList = new List ();
// This loop will iterate on the List PaidInvoiceNumberList and will process each record
for (APEX_Invoice__c objInvoice: PaidInvoiceNumberList) {
// Condition to check the current record in context values
if (objInvoice.APEX_Status__c == 'Paid') {
// if Status value is paid then it will update the paid date to systems today's date
objInvoice.Paid_Date__c = System.today();
// current record on which loop is iterating
System.debug('Value of Current Record on which Loop is iterating is'+objInvoice);
// if Status value is paid then it will the invoice number into List of String
InvoiceNumberList.add(objInvoice);
}
}
if(InvoiceNumberList.size() > 0) {
Update InvoiceNumberList;
}
Decoding of Apex:
// SOQL query which will fetch the invoice records which has been created today
PaidInvoiceNumberList = [SELECT Id,Name, APEX_Status__c,Paid_Date__c FROM APEX_Invoice__c WHERE CreatedDate = System.today()];
We will have to get invoice records from the Invoice object with a condition as mentioned in SOQL query. Drag the “Get Records” element into the canvas and give information accordingly. As we are getting all the records, flow will automatically create a record collection and store all field values or just like in the above SOQL query you can choose specific fields. I choose Automatically store all fields.

// This loop will iterate on the List PaidInvoiceNumberList and will process each record
for (APEX_Invoice__c objInvoice: PaidInvoiceNumberList) {
// Condition to check the current record in context values
if (objInvoice.APEX_Status__c == 'Paid') {
// if Status value is paid then it will update the paid date to systems today's date
objInvoice.Paid_Date__c = System.today();
// current record on which loop is iterating
System.debug('Value of Current Record on which Loop is iterating is'+objInvoice);
// if Status value is paid then it will the invoice number into List of String
InvoiceNumberList.add(objInvoice);
}
In this block of code they are initiating a loop and checking each record in the loop where status is “Paid.” Let’s see how it is done in flow.
First we have the Loop element and define record collection variable and loop variable which hold a single record at a time.
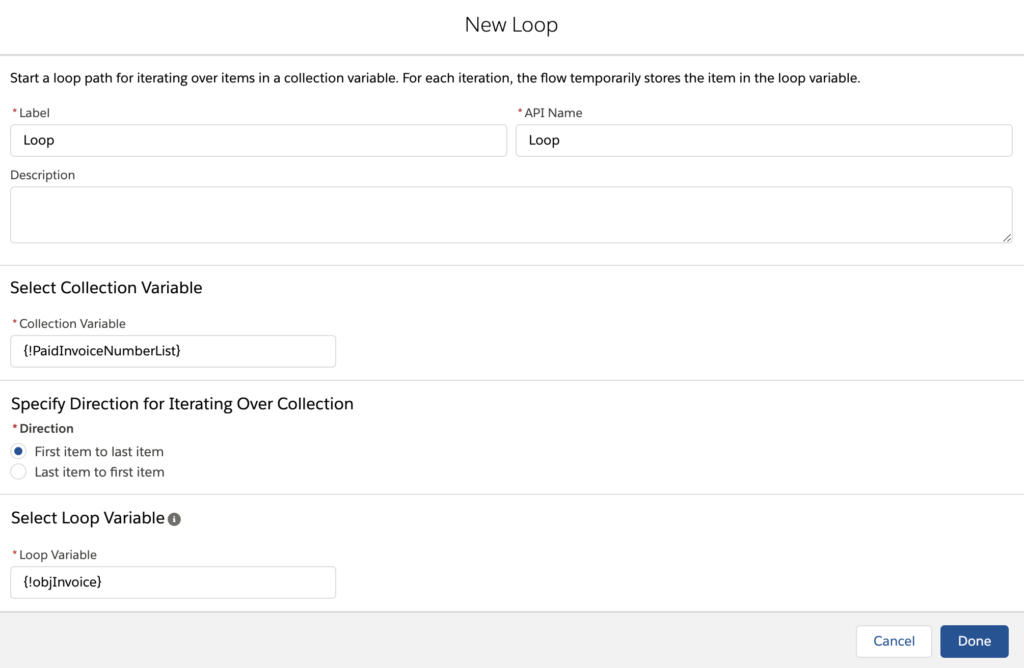
Now, we will have a Decision element to check for records with status.
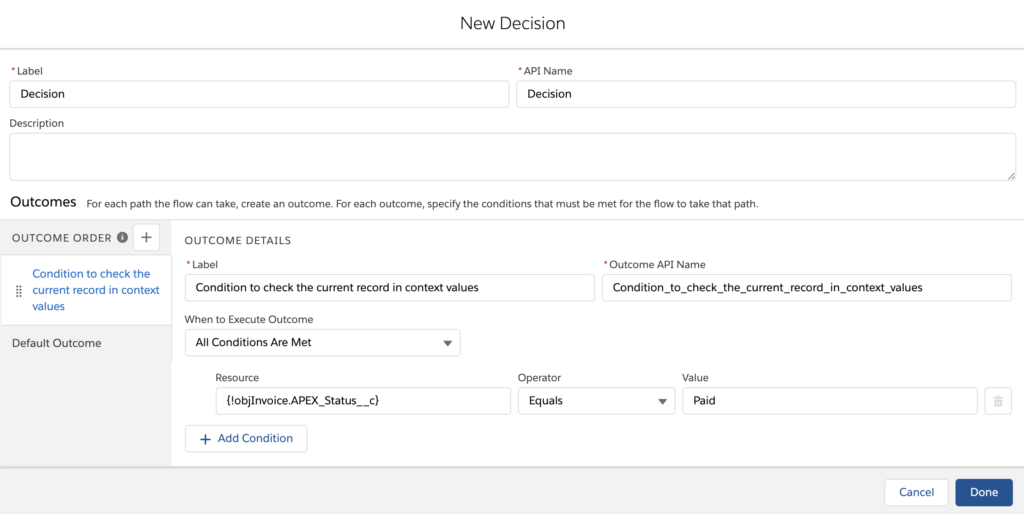
// if Status value is paid then it will update the paid date to systems today's date
objInvoice.Paid_Date__c = System.today();
// if Status value is paid then it will the invoice number into List of String
InvoiceNumberList.add(objInvoice);
For the records where if condition is satisfied status is equal to paid are updated with paid date field equals to today’s date and add the records to a list called “InvoiceNumberList” here these will be a record collection resource. Let’s see how this is executed in flow.
First we have to update the Paid Date field to today’s date and we have to create a resource “InvoiceNumberList” record collection and then use assignment to add the records which meet status paid into the collection.
Now we have to update the list as per the code, it is checking if the list size is greater than zero or not, in flow you can check if the list is not null.
if(InvoiceNumberList.size() > 0) {
Update InvoiceNumberList;
}
Drag & drop the Decision element.
Now drag & drop the Update Records element, In this element you can select the whole list and update the records.
Now lets see how flow looks.
System.debug('Value of Current Record on which Loop is iterating is'+objInvoice);
Just like in Apex code where you can debug. Same feature is available in flows, before activating the flow i recommend you to debug your flow.
Conclusion:
Hope this give’s good understanding of Salesforce flows. Will meet you guys next time with another topic.